CASA ȘTIINȚEI
CASA ȘTIINȚEI este locul unde diverse subiecte sunt dezbătute și scrise.
|
Lista Forumurilor Pe Tematici
|
CASA ȘTIINȚEI | Inregistrare | Login
POZE CASA ȘTIINȚEI
Nu sunteti logat.
|
Nou pe simpatie: iris22
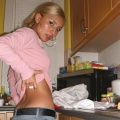 | Femeie 23 ani Bucuresti cauta Barbat 32 - 63 ani |
|
blurex
Īnvățăcel
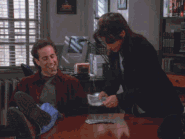 Inregistrat: acum 8 ani
Postari: 97
|
|
Sursa:
Code:
<script>
aici intra codul JavaScript in HTML pentru o
</script> |
Code:
<script src="JavaScript.js">
/* incarca codul dintr-un fisier */
</script> |
================================ variabile
Code:
document.write("Salut lumea mea!");
var years = 35;
var someText = " years old ";
var gameOver = true;
alert (years + someText + gameOver); //afiseaza 35 years old true |
================================= operatori
Code:
var number1 = 7;
var number2 = 4;
number1 += number2;
number1++;
document.write (number1); |
//afiseaza 12
================================== funcții
Code:
function addSomething (num, str)
{
var add = num + str;
alert (add);
}
addSomething (455, " Programs to write"); //afiseaza "455 Programs to write" |
=============================== condiții
Code:
var food = "Apples";
var mancare = "suficienta";
if(food == "Apple" && mancare != "nimic")
{
alert("We ate an Apple");
}
else if(food == "Meat" || mancare == "nimic")
{
alert("We did not eat an Apple, but we ate Meat");
}
else
{
alert("Nebuine ai mancat!"); //afiseaza nebunie ai mancat
} |
============================= loops
Code:
/*
var i = 1;
while(i < 5)
{
document.write("A statement has run");
i++;
}
*/
for(var i = 1; i < 5; i++)
{
document.write("A statement has run ");
} |
================================= return
Code:
function add(x,y)
{
result = x * y;
return result;
}
var theResult = add(6,5);
document.write(theResult); |
==================================== global and local variables
Code:
var name = "Ana"; //variabila globala
function printName()
{
name2 = "Sandu"; //variabila globala
var name3 = "Petrica"; //variabila locala
}
printName(); //se apeleaza functia, daca nu s-ar apela, n-ar aparea name2
document.write(name2); //afiseaza "Sandu"
document.write(name3); //nu afiseaza nimic pentru ca este variabila locala |
======================================= Pass by value
Code:
function battin(player, distance)
{
var more = player + " hit the ball " + distance + " feet";
document.write(more);
}
battin("Steve", 351); //afiseaza "Steve hit the ball 351 feet" |
Nu "Steve", 351 sunt transmise functiei, ci programul copiaza valorile si le trimite functiei.
======================================= Arrays
Code:
var roads = ["stone", "dirt", "cement", "tar"];
var roads[4] = "gravel";
var roadTravelled = roads[1];
document.write(roadTravelled); //afiseaza "dirt" |
======================================== objects
Se separa prin virgula precum vectorii
Code:
var orc =
{
color: "green",
height: 5,
weight: 180,
yell: function()
{
document.write("Orcs are the best");
}
};
orc.yell(); //afiseaza "Orcs are the best"
orc.hair = "red";
delete orc.hair;
document.write(orc.hair); //afiseaza "undefined" |
Functions inside objects are called methods
================================== The String Objects
Code:
var hello = "Hello";
//hello = hello.length; //afiseaza
//hello = hello.toUpperCase();
//hello = hello.charAt(4); //afiseaza "o"
//hello = hello.replace('Hello', 'Today'); //afiseaza "Today"
hello = hello.bold();
hello = hello.italics();
document.write(hello); |
==================================== The Math Objects
Code:
var number = 4.7;
var newNumber = 26 + Math.round(number); //rotunjeste nr.
var newNumber = 26 + Math.ceil(number); //rotunjeste catre urmatorul numar intreg "5"
var newNumber = 26 + Math.floor(number); //rotunjeste catre partea intreaga "4"
var number = Math.sqrt(16); //face radical din 16 (=4)
document.write(newNumber); |
==================================== The Date Objects
Code:
var todayDate = new Date();
todayDate.setYear(1785); //modifica anul
document.write(todayDate);
var useString = todayDate.toDateString(); //arata doar data
document.write(useString);
//datele sun scoase din systemclock |
====================================== The DOM - Document Object Model
1. Browser loads page 2. DOM is created - objects that we can use that represents the HTML. Objects are stored in the DOM.
The DOM allows us to use our JavaScript code to acces parts of the web page.
<p id="ceva">Textul aici</p>
1. Element node (<p></p>) 2. Attribute node (id="ceva") 3. Text node ("Textul aici")
1. Need to grab the element ID. We can do that with the getelementbyid method. 2. Once we have the ID we are good to go and we can start to style the element with the style object which is another object in the DOM.
CSS JavaScript --- ---------- color color border border margin margin
background-color backgroundColor font-style fontStyle text-decoration textDecoration
padding-left padding-left border-left border-left
================================
HTML
Code:
<!DOCTYPE html>
<html>
<head>
<script src="js.js">
</script>
</head>
<body>
<p id="para1">Text pentru milionari </p>
<button onclick="changeStyle()">Submit</button>
</body>
</html> |
JavaScript
Code:
function changeStyle()
{
//var text = document.getElementById("para1").style.color = "blue";
//var text = document.getElementById("para1").style.backgroundColor = "blue";
var text = document.getElementById("para1").style.fontStyle = "italic";
} |
===================================
Code:
getElementsByTagName |
HTML
Code:
<!DOCTYPE html>
<html>
<head>
<script src="js.js">
</script>
</head>
<body>
<p id="para1">Text pentru milionari </p>
<p id="para2">Text pentru milionari </p>
<p id="para3">Text pentru milionari </p>
<p id="para4">Text pentru milionari </p>
<button onclick="changeStyle()">Submit</button>
</body>
</html> |
JavaScript
Code:
function changeStyle()
{
var paragraph = document.getElementsByTagName("p");
var changeParaText = paragraph[1].style.fontStyle = "italic"; //schimba doar elementul al doilea in Italice
} |
Code:
function changeStyle()
{
var paragraph = document.getElementsByTagName("p");
for(var i = 0; i < paragraph.length; i++)
{
paragraph[i].style.fontStyle = "italic" //face toate paragrafele cu italic
}
} |
==================================
Code:
getElementsByClassName |
ATENTIE: getElementsByClassName[0], getElementsByClassName[1], ... reprezinta elementele numerotate din clasa in ordinea aparitei lor
HTML
Code:
<!DOCTYPE html>
<html>
<head>
<script src="js.js">
</script>
</head>
<body>
<p class="para">Salutari multe </p>
<p class="para">din lumea milionarilor</p>
<p class="para"></p>
<button onclick="changeStyle()">Alt text</button>
</body>
</html> |
JavaScript
Code:
function changeStyle()
{
var paragraph = document.getElementsByClassName("para");
var firstParaText = paragraph[0].innerHTML;
var secondParaText = paragraph[1].innerHTML;
var addThem = paragraph[2].innerHTML = firstParaText + secondParaText;
var firstParaText = paragraph[0].innerHTML = " ";
var secondParaText = paragraph[1].innerHTML = " ";
} |
In programul de mai sus se foloseste si
Cu innerHTML cele doua paragrafe din HTML sunt concatenate in paragraph[2] iar [0] si [1] primesc o valoare " " ca sa dispara.
=================================================================================
HTML
Code:
<!DOCTYPE html>
<html>
<head>
<script src="js.js">
</script>
</head>
<body>
<p class="para">Salutari multe</p>
<img src="ion.jpg" id="image"/> <br><br>
<button onclick="changeStyle()">Schimba imaginea</button>
</body>
</html> |
JavaScript
Code:
function changeStyle()
{
document.getElementById("image").src="corneliu.jpg";
} |
Schimbă imaginea cand se face click pe ea.
===================================================================================
1. Choose the element where we will place our event (in this case the button element) 2. Choose the event we want to use (in this case onclick)
aici am ramas
Modificat de blurex (acum 8 ani)
|
|
pus acum 8 ani |
|